Response Areas
TabSINT comes with many different types of response areas that can be used within protocols. Select one of the following response areas for a protocol example and image of each response area type.
Audiometry Input Response Area
Controls which fields the user will be prompted for in audiometry tests as well as the audiogram frequencies used.
Protocol Example
{
"id": "Audiometry",
"title": "Audiometry Input Response Area Example",
"responseArea": {
"type": "audiometryInputResponseArea",
"fieldsToSkip": {
"skipGender": true
}
}
}
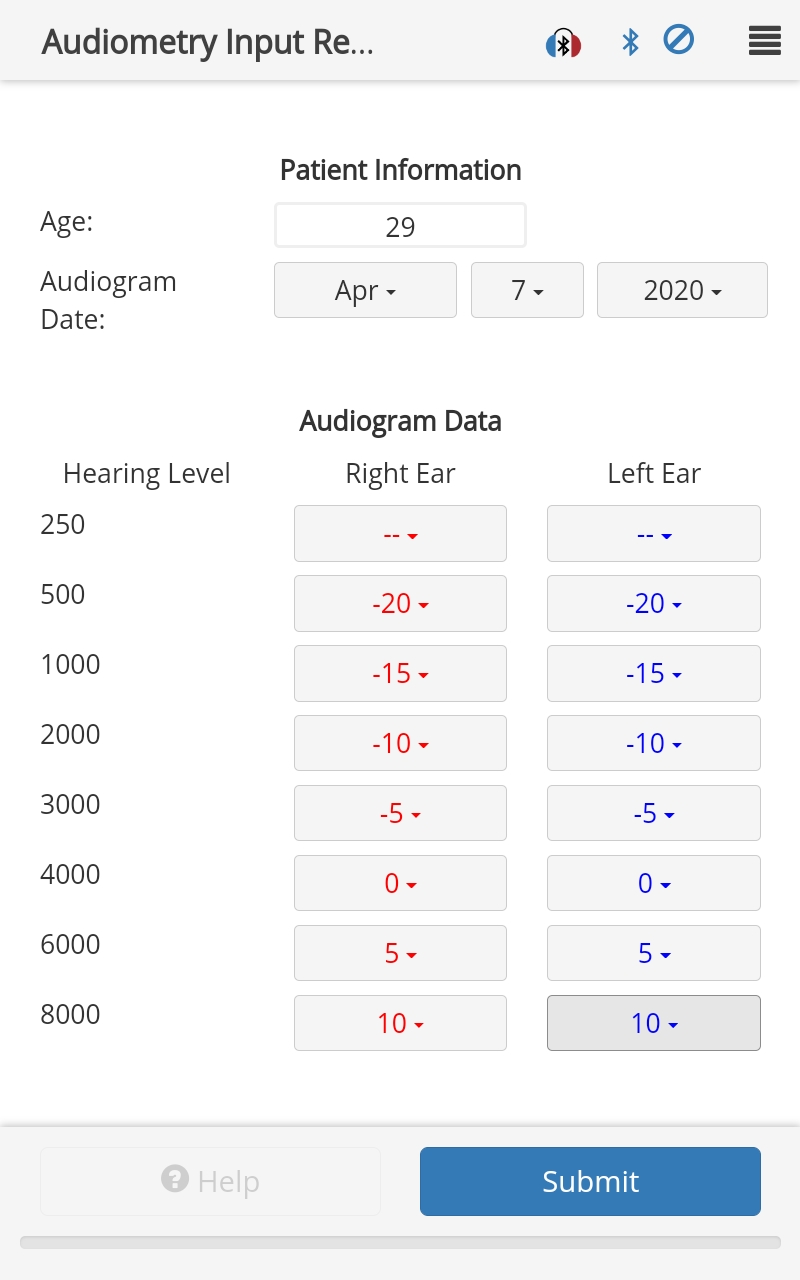
Options
fieldsToSkip
:Type:
object
Description: Object that can contain the following properties:
skipAge
:- Type:
boolean
- Description: Skips age input if
true
.
- Type:
skipGender
:- Type:
boolean
- Description: Skips gender input if
true
.
- Type:
skipDate
:- Type:
boolean
- Description: Skips date input if
true
.
- Type:
audiogramFrequencies
:- Type:
array
- Description: Array of audiogram frequencies to poll the user for. (Default =
[250, 500, 1000, 2000, 3000, 4000, 6000, 8000]
)
- Type:
Response
The result.response
from an audiometryInputResponseArea
is an object with multiple items. Each item is a string. For the example shown here:
result.response.Age = "29" // Note this is a string.
result.response.Audiometry Month = "Jan"
result.response.Audiometry Day = "28"
result.response.Audiometry Year = "2020"
Because skipGender
was set to true
there is no result.response.Gender
.
Two objects, left
and right
, contain hearing levels for the frequencies at which a response was specified. This example used the default set of frequencies, but no hearing level was specified at 250 Hz. Therefore, no response object is generated for that frequency. The responses given are:
result.response.right.500 = "-20"
result.response.right.1000 = "-15"
result.response.right.2000 = "-10"
result.response.right.3000 = "-5"
result.response.right.4000 = "0"
result.response.right.6000 = "5"
result.response.right.8000 = "10"
result.response.left.500 = "-20"
result.response.left.1000 = "-15"
result.response.left.2000 = "-10"
result.response.left.3000 = "-5"
result.response.left.4000 = "0"
result.response.left.6000 = "5"
result.response.left.8000 = "10"
Schema
Button Grid Response Area
Allows choices between an arbitrary number of radio button choices in a grid.
Protocol Example
{
"id": "Button Grid",
"title": "Button Grid Response Area Example",
"questionMainText": "Select your answer",
"responseArea":{
"type": "buttonGridResponseArea",
"feedback": "showCorrect",
"rows": [
{
"choices":[
{
"id":"One",
"correct": true
},
{
"id":"Two"
},
{
"id":"Three"
}
]
},
{
"choices": [
{
"id":"Four"
},
{
"id":"Five"
},
{
"id":"Six"
}
]
},
{
"choices": [
{
"id":"Seven"
},
{
"id":"Eight"
},
{
"id":"Nine"
}
]
}
]
}
}
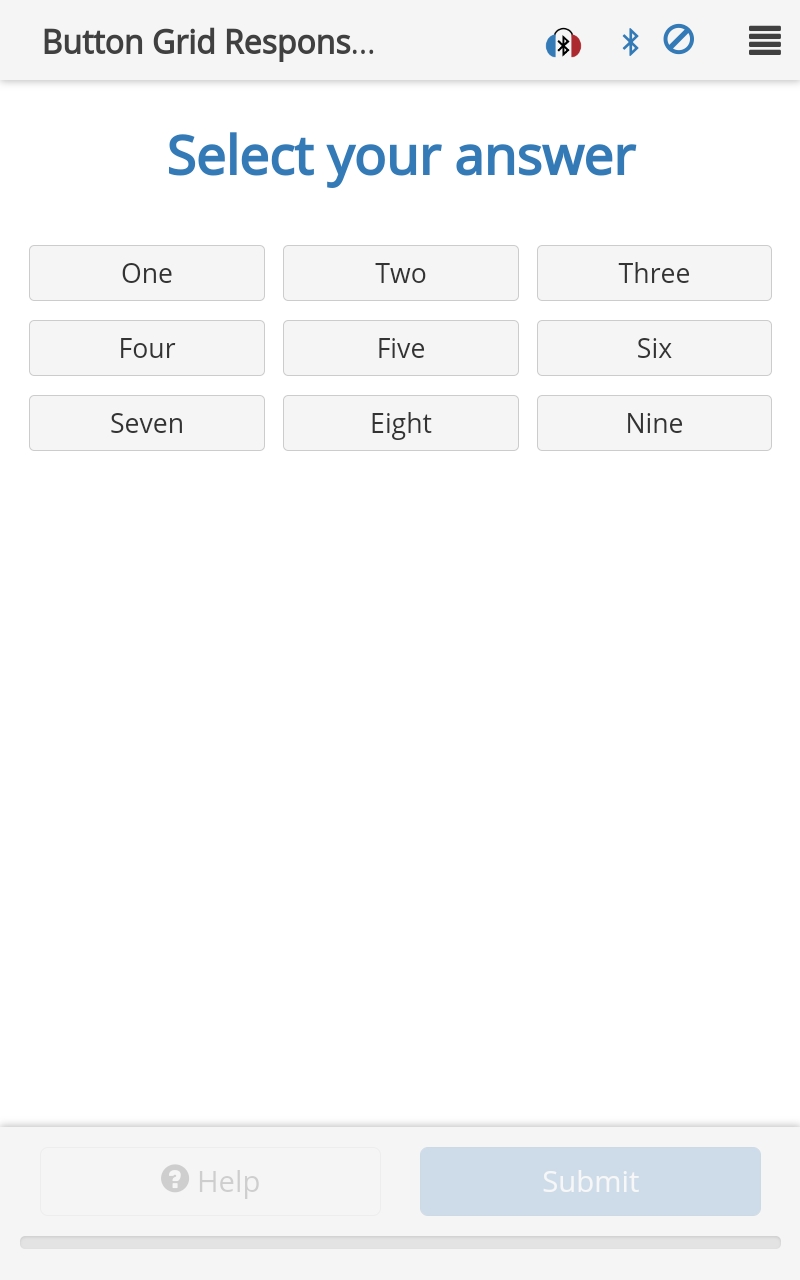
Options
feedback
:- Type:
string
- Description: Options are
gradeResponse
andshowCorrect
.
- Type:
rows
:- Type:
array
- Description: Each object within the array defines a row. Each object has a choice array. Each object within the
choices
array defines the columns in that row.
- Type:
verticalSpacing
:- Type:
integer
- Description: Vertical spacing between buttons, given in [px].
- Type:
hortizontalSpacing
:- Type:
integer
- Description: Horizontal spacing between buttons, given in [px].
- Type:
delayEnable
:- Type:
integer
- Description: Delay (ms) before the buttons are active to accept a response. (Default=0)
- Type:
Response
The result.response
from a buttonGridResponseArea
is a string containing the id
of the selected button. If the user selected the button choice with id
of One
(note that the text
displayed on the button is not necessarily the same as the id
of the button), then:
result.response = "One"
Additionally, if one of the options is identified as correct
within the page definition, result.correct
will indicate if the user responded correctly. For the example above:
result.correct = true
Schema
Checkbox Response Area
Allows user to select more than one choice using checkbox controls.
Protocol Example
{
"id": "Checkbox Response Area",
"title": "Checkbox Example",
"questionMainText": "What is your favorite color?",
"questionSubText": "Confirm that you can choose none or more than one and submit.",
"responseArea": {
"type": "checkboxResponseArea",
"choices": [
{
"id": "Red"
},
{
"id": "Blue"
},
{
"id": "Green"
}
],
"other" : "Some other color"
}
}
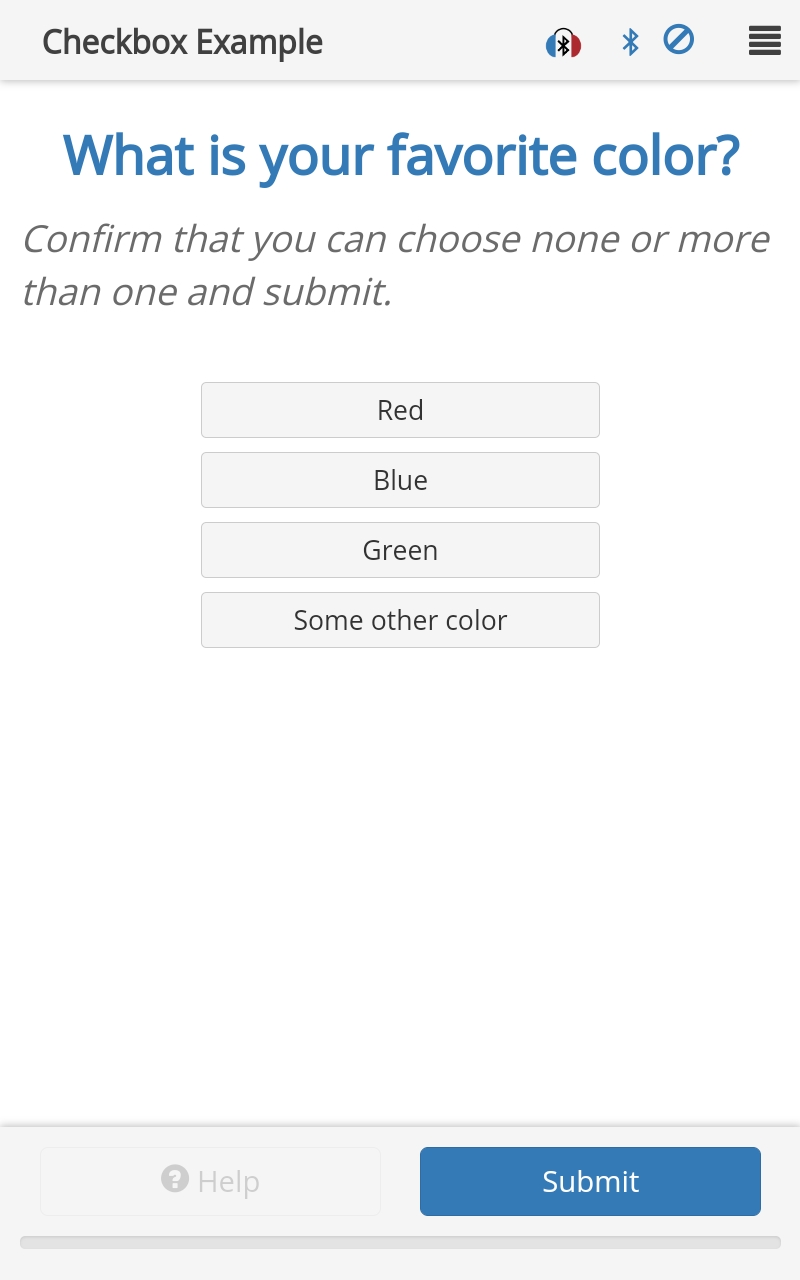
Options
choices
:- Type:
array
- Description: Array of objects containing the choice properties.
- Type:
buttonScheme
:- Type:
string
- Description: Can be
markIncorrect
(where green indicates not selected and red indicates selected), ormarkCorrect
(where green indicates the correct item was selected and red indicates that the correct item was not selected). Default is standard checkbox behavior.
- Type:
other
:- Type:
string
- Description: Text label for an 'other' choice. E.g.,
Other
, orSomewhere else
. If selected, the user will be prompted for an optional text value which will be stored in anotherResponse
field in the result object. If this field is not defined, theOther
choice is not presented.
- Type:
verticalSpacing
:- Type:
integer
- Description: Vertical spacing between buttons, given in [px].
- Type:
Response
The result.response
from an checkboxResponseArea
is an array of strings. In the protocol example above, if the user selects "Red" and "Blue":
result.response = "["Red", "Blue"]" // Note this is a string.
When using the response in a
followOn
orflag
, a protocol developer needs to deserialize theresult.response
string to use list response. For example,
// This will return true for the example above JSON.parse(result.response).includes("Blue");
Schema
Custom Response Area
Allows for a custom javascript file and associated custom html file to define the response area. See the custom response areas documentation for details regarding the javascript and html files.
Protocol Example
{
"id": "Custom",
"title": "Custom HTML and JS",
"questionMainText": "Custom Response Area",
"responseArea": {
"type": "customResponseArea",
"html": "customHtml.html"
}
}
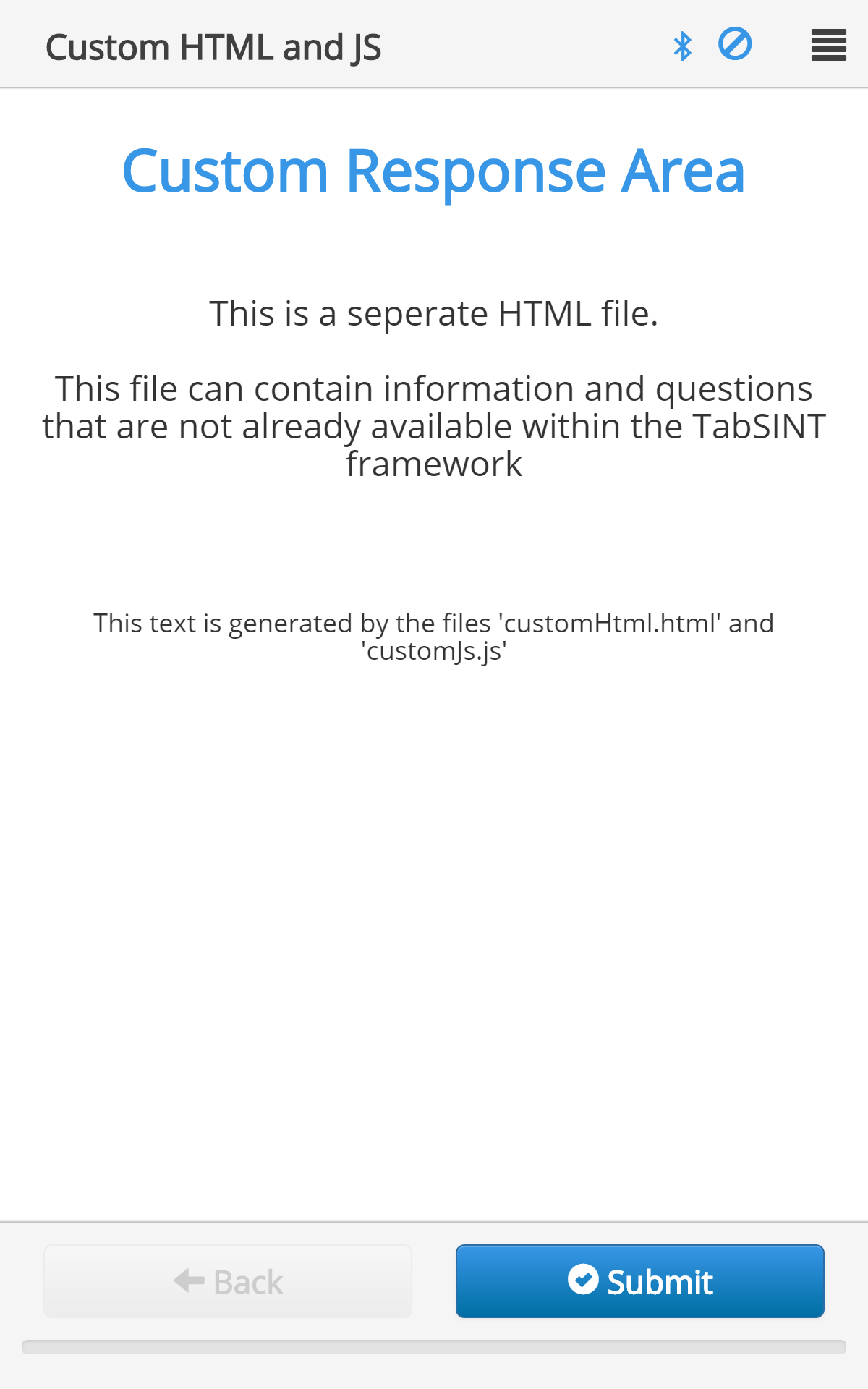
Options
html
:- Type:
string
- Description: Filename of the custom html file. (e.g.
customHtml.html
)
- Type:
js
:- Type:
string
- Description: Filename of the custom javascript file. (e.g.
customJs.js
)
- Type:
module
:- Type:
string
- Description: Module name for the custom javascript file. (e.g.
customJs
)
- Type:
Schema
Image Map Response Area
Allows the user to select a location within an image.
Protocol Example
{
"id":"imagemap001",
"questionMainText": "Pick your Favorite Gnome",
"questionSubText": "You can skip this page.",
"responseArea": {
"type":"imageMapResponseArea",
"enableSkip": true,
"image": {
"path": "gnomes.jpg"
},
"hotspots": [
{
"id": "gnome1",
"shape": "rect",
"coordinates": "7,20,22,42"
},
{
"id": "gnome2",
"shape": "rect",
"coordinates": "42,15,56,38"
},
{
"id": "gnome3",
"shape": "rect",
"coordinates": "75,17,90,43"
}
]
}
}
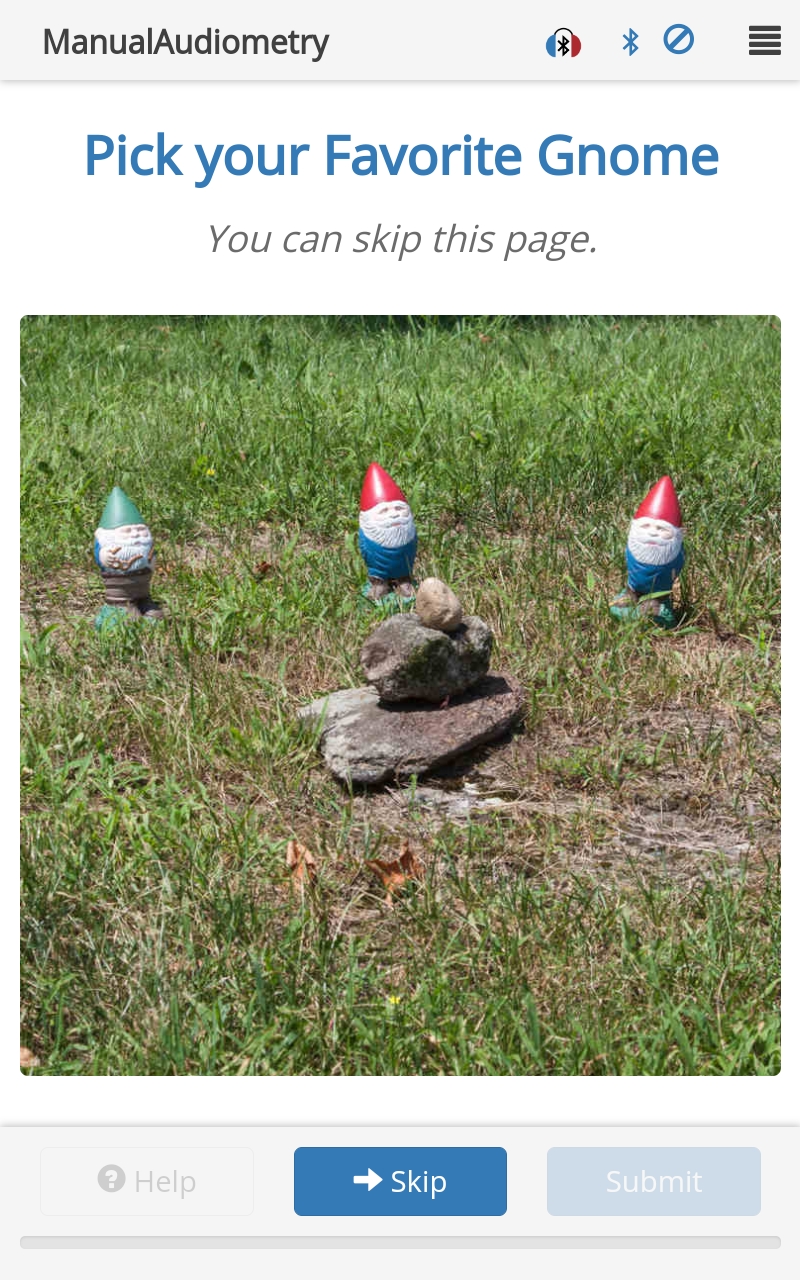
Options
image
:Type:
object
Description: An object containing the properties:
path
:- Type:
string
- Description: Filename of image. Required.
- Type:
width
:- Type:
string
- Description: Width of image, e.g.,
80%
. (Default =100%
)
- Type:
hotspots
:Type:
array
Description: An array of objects defining the active clickable locations within the image map. Each object can contain:
id
:- Type:
string
- Description: ID of the hotspot. Required.
- Type:
shape
:- Type:
string
- Description: Shape of the hotspot within the image. Can be
circle
,rect
,poly
, ordefault
.
- Type:
coordinates
:- Type:
string
- Description: Coordinates of the hotspot in the picture, referenced to the top-left of the image. Note that coordinates defined later will overwrite earlier coordinates. For each
shape
:circle
: [X0%, Y0%, Radius%] (where radius % is relative to image width)rect
: [X0% Y0% X1% Y2%]poly
: [X0% Y0%...Xn% Yn%]default
: sets all area within the image that hasn't already been specified
- Type:
correct
:- Type:
boolean
- Description: If
true
, set the hotspot to the correct answer.
- Type:
other
:- Type:
boolean
- Description: If
true
, another
text input will show up below the image when the hotspot is clicked.
- Type:
Response
The result.response
from an imageMapResponseArea
is a string corresponding to the id
of the selected hotspot. In the example here, if the user were to select the left-most gnome the result would be:
result.response = "gnome1"
Schema
Integer Response Area
The integer response area allows the input of a number.
Protocol Example
{
"id": "Input a number",
"title": "Numeric Response Area Example",
"questionMainText": "Input your age",
"responseArea": {
"type":"integerResponseArea",
"responseRequired": true
}
}
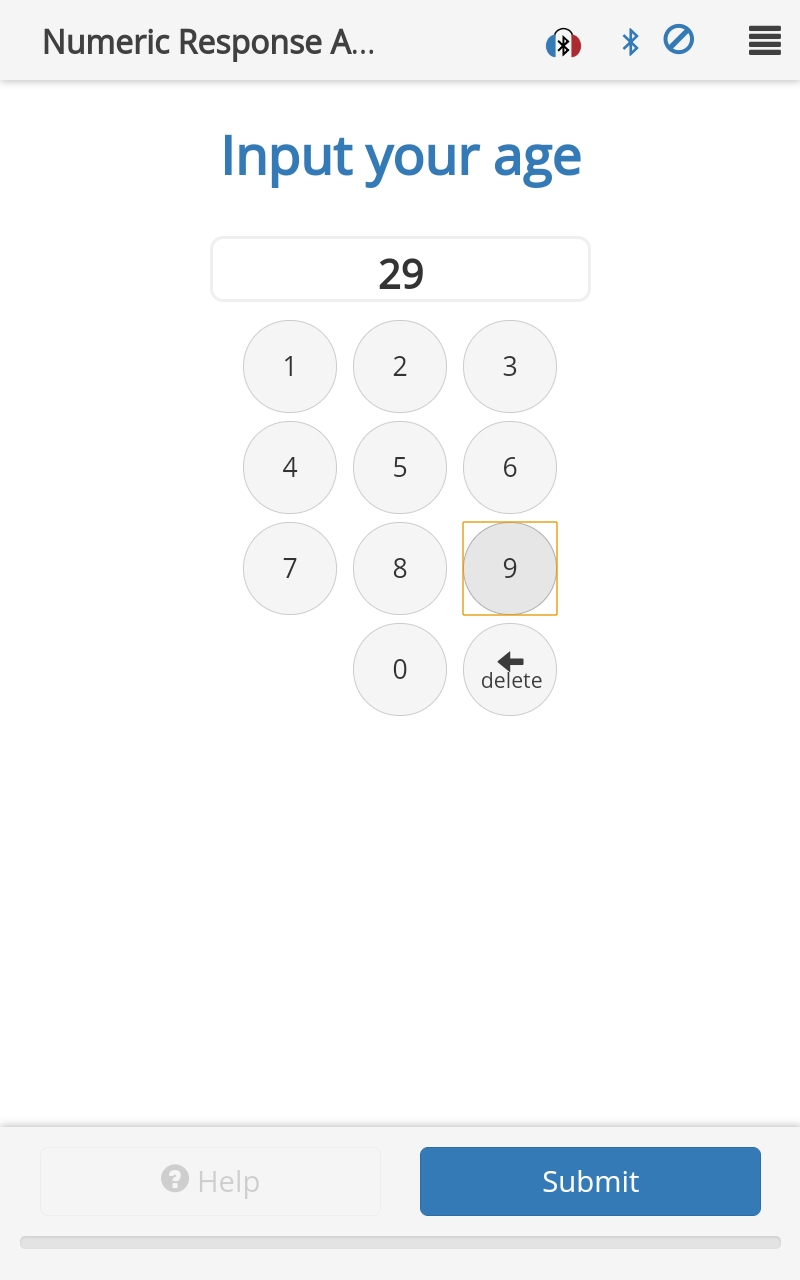
Options
minAllowedValue
:- Type:
number
- Description: The page cannot be submitted unless the entered value is greater than or equal to this number. If not defined, no minimum is enforced.
- Type:
maxAllowedValue
:- Type:
number
- Description: The page cannot be submitted unless the entered value is less than or equal to this number. If not defined, no maximum is enforced.
- Type:
float
:- Type:
boolean
- Description: If
true
, converts the integer response area into a floating point response area, including a key to add a decimal.
- Type:
negative
:- Type:
boolean
- Description: If
true
, allows for a negative number to be entered.
- Type:
value
:- Type:
number
- Description: Prepopulate the input box with this value. If not defined, the input box is not prepopulated.
- Type:
Response
The result.response
from an integerResponseArea
is a string representation of the number entered by the user. If the user enters the number 29, then:
result.response = "29" // Note this is a string.
Schema
Likert Response Area
A Likert scale answer, with optional specifiers for some or all of the points.
Protocol Example
{
"id": "likert_004",
"title": "Likert",
"questionMainText": "It was easy for the person to put the headset on me.",
"instructionText": "Test Cases 004, 005, 201 and 202",
"responseArea": {
"type": "likertResponseArea",
"exportToCSV": true,
"levels": 5,
"useEmoticons": true,
"specifiers": [
{
"level": 0,
"label": "Strongly Disagree"
},
{
"level": 1,
"label": "Disagree"
},
{
"level": 2,
"label": "No Opinion"
},
{
"level": 3,
"label": "Agree"
},
{
"level": 4,
"label": "Strongly Agree"
}
]
}
},
{
"id": "likert_005",
"title": "Likert",
"instructionText": "Test Cases 203, 204, 205",
"responseArea": {
"type": "likertResponseArea",
"exportToCSV": true,
"questions": [
{
"questionMainText": "Did you have any trouble hearing soft sounds before being exposed to noise?",
"levels": 11,
"labelFontSize": 15,
"specifiers": [
{
"level": 0,
"label": "No trouble",
"position": "below"
},
{
"level": 0,
"label": "0",
"position": "above"
},
{
"level": 1,
"label": "1"
},
{
"level": 2,
"label": "2"
},
{
"level": 3,
"label": "3"
},
{
"level": 4,
"label": "4"
},
{
"level": 5,
"label": "5"
},
{
"level": 6,
"label": "6"
},
{
"level": 7,
"label": "7"
},
{
"level": 8,
"label": "8"
},
{
"level": 9,
"label": "9"
},
{
"level": 10,
"label": "Great deal of trouble",
"position": "below"
},
{
"level": 10,
"label": "10",
"position": "above"
}
]
},
{
"questionMainText": "Do you have any trouble hearing soft sounds RIGHT NOW?",
"levels": 11,
"labelFontSize": 30,
"specifiers": [
{
"level": 0,
"label": "No trouble",
"position": "above"
},
{
"level": 0,
"label": "0",
"position": "below"
},
{
"level": 1,
"label": "1",
"position": "below"
},
{
"level": 2,
"label": "2",
"position": "below"
},
{
"level": 3,
"label": "3",
"position": "below"
},
{
"level": 4,
"label": "4",
"position": "below"
},
{
"level": 5,
"label": "5",
"position": "below"
},
{
"level": 6,
"label": "6",
"position": "below"
},
{
"level": 7,
"label": "7",
"position": "below"
},
{
"level": 8,
"label": "8",
"position": "below"
},
{
"level": 9,
"label": "9",
"position": "below"
},
{
"level": 10,
"label": "Great deal of trouble",
"position": "above"
},
{
"level": 10,
"label": "10",
"position": "below"
}
]
}
]
}
},
{
"id": "backtomain",
"reference":"MainMenu"
}
]
}
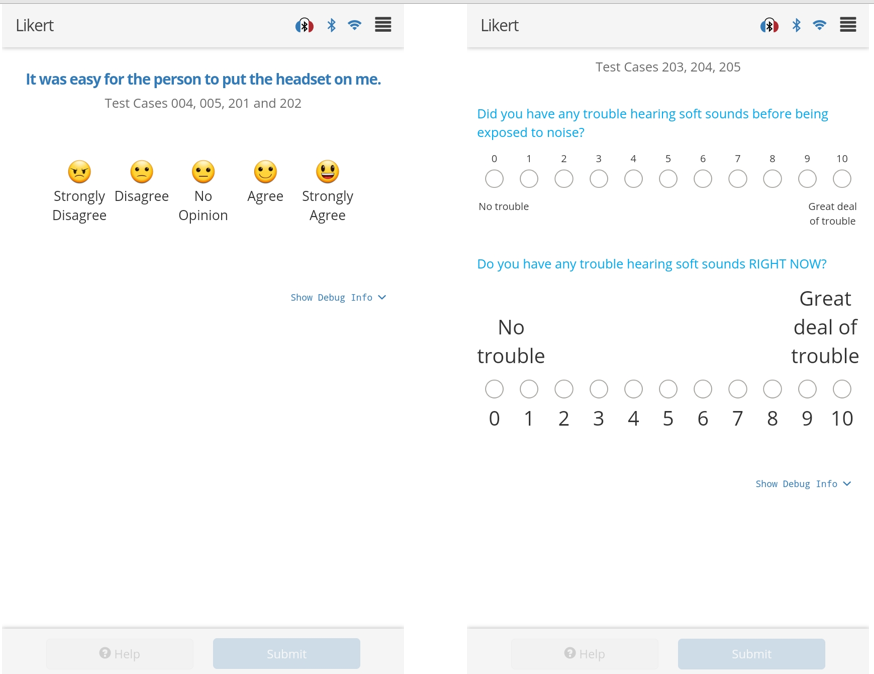
Options
autoSubmit
:- Type:
boolean
- Description: If
true
, go straight to next page when a choice is selected. (Default =false
)
- Type:
useEmoticons
:- Type:
boolean
- Description: If
true
, use emoticons instead of radio buttons. Requires levels == 5. (Default =false
)
- Type:
levels
:- Type:
number
- Description: Integer value representing how many levels (i.e. if
3
, then the choices are 0, 1, and 2).
- Type:
centerLabelAbove
:- Type:
string
- Description: Label that is above the Likert scale and centered to indicate that it applies to all
above
Likert labels.
- Type:
centerLabelBelow
:- Type:
string
- Description: Label that is below the Likert scale and centered to indicate that it applies to all
below
Likert labels.
- Type:
labelFontSize
:- Type:
number
- Description: Override font size of specifier labels, in px. (Default =
20
)
- Type:
specifiers
:Type:
array
Description: Array containing the following objects:
level
:- Type:
number
- Description: An integer specifying the level. Required.
- Type:
label
:- Type:
string
- Description: Label to associate with the level. Required.
- Type:
position
:- Type:
string
- Description: The location of the label, either
above
orbelow
. (Default =above
)
- Type:
questions
:Type:
array
Description: Each object within this array defines an individual question. Each object can contain:
questionMainText
:- Type:
string
- Description: Primary (large) text centered on the page. Overrides the page
questionMainText
.
- Type:
useEmoticons
:- Type:
boolean
- Description: If
true
, use emoticons instead of radio buttons. Requires levels == 5. (Default =false
)
- Type:
levels
:- Type:
number
- Description: Integer value representing how many levels (i.e. if
3
, then the choices are 0, 1, and 2).
- Type:
centerLabelAbove
:- Type:
string
- Description: Label that is above the Likert scale and centered to indicate that it applies to all
above
Likert labels.
- Type:
centerLabelBelow
:- Type:
string
- Description: Label that is below the Likert scale and centered to indicate that it applies to all
below
Likert labels.
- Type:
labelFontSize
:- Type:
number
- Description: Override font size of specifier labels, in px. (Default =
20
)
- Type:
specifiers
:Type:
array
Description: Array containing the following objects:
level
:- Type:
number
- Description: An integer specifying the level. Required.
- Type:
label
:- Type:
string
- Description: Label to associate with the level. Required.
- Type:
position
:- Type:
string
- Description: The location of the label, either
above
orbelow
. (Default =above
)
- Type:
exportToCSV
:- Type:
boolean
- Description: If
true
, export the result to CSV upon submitting exam results. (Default =false
)
- Type:
Response
The result.response
from a likertResponseArea
is a string array where the number of array elements corresponds to the number of questions presented. For each element, the value of the string is equal to the 0-based level selected. In this example, if the user selected "Agree" to the first question and "No Opinion" to the second then the result would be:
result.response = ["3","2"]
Schema
MPANL Response Area
A response area for measuring Maximum Permissible Ambient Noise Levels (MPANL) with a Svantek dosimeter.
Protocol Example
{
"id": "MPANLs",
"title": "MPANLs",
"headset": "WAHTS",
"questionMainText": "Svantek Background Noise Measurement",
"instructionText": "Press 3 or 10 seconds and then wait quietly for measurement to complete. Repeat the measurement if necessary.",
"responseArea": {
"type": "mpanlResponseArea"
}
}
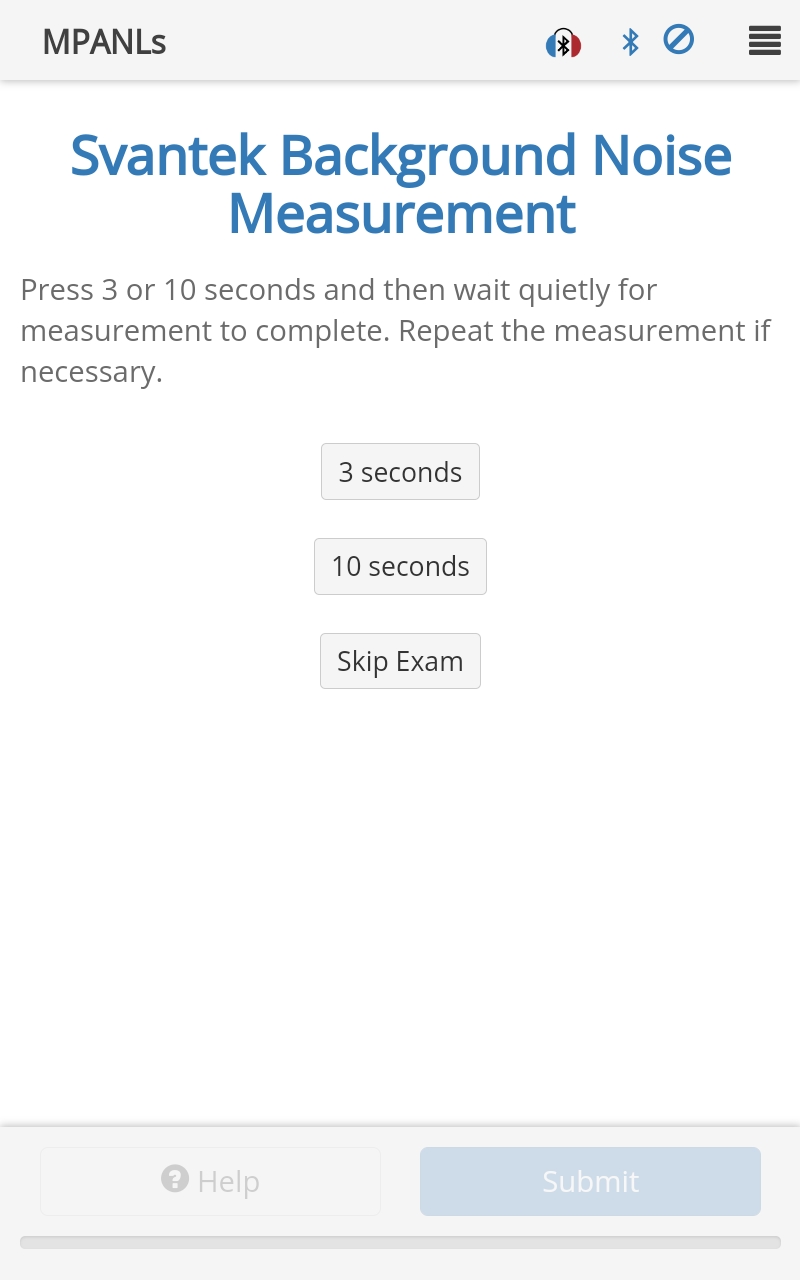
Options
autoSubmit
:- Type:
boolean
- Description: If
true
, go straight to next page once this page is complete. (Default =false
)
- Type:
standard
:- Type:
string
- Description: The octave band levels standard to compare to. Can be
ANSI S3.1-R2008
,DoD
orOSHA
. (Default =ANSI S3.1-R2008
)
- Type:
F
:- Type:
array
- Description: Report levels at these frequencies (Hz). Default =
[125, 250, 500, 1000, 2000, 4000, 8000]
.
- Type:
MPANL
:- Type:
array
- Description: Specify maximum permissible ambient noise levels (MPANL) instead of using those provided by the
standard
. Array length must match the length ofF
.
- Type:
attenuation
:- Type:
array
- Description: Specify the headset attenuation (in dB) at
F
frequencies. By default, TabSINT will look at theheadset
property of the protocol or page to define the attenuation. Array length must match the length ofF
.
- Type:
exportToCSV
:- Type:
boolean
- Description: If
true
, export the result to CSV upon submitting exam results. (Default =false
)
- Type:
Response
The result.response
from an mpanlResponseArea
has two possible values, either "continue" or "skipped". If the user selected either 3 seconds or 10 seconds, then:
result.response = "continue" // Note this is a string.
Additional result fields contain the Svantek data:
result.duration = 3000 // length of measurement (ms)
result.svantek.time = "2020-05-15T19:04:20.214Z" // time the measurement was made
result.svantek.status = 40 // success status code from the Svantek
result.svantek.Leq = [43, 41, ...] // array of measured third-octave band levels
result.svantek.Frequencies = [20, 25, ..., 10000] // array of third-octave band frequencies
result.svantek.LeqA = 41.52 // A-weighted equivalent level
result.svantek.LeqC = 50.38 // C-weighted equivalent level
result.svantek.LeqZ = 55.06 // Z-weighted equivalent level
result.svantek.FBand = [ 125, 250, ..., 8000] // array of frequencies defined as F on protocol page
result.svantek.bandLevel = [ 41.9, 40.3, ..., 48.5] // array of levels corresponding to FBand frequencies
The result.mpanlsData
is an array of objects where each object corresponds to a frequency in result.svantek.FBand
and contains the following information:
result.mpanlsData[0].freq = 125
result.mpanlsData[0].level = "41.9" // level measured at this frequency (dB SPL)
result.mpanlsData[0].limit = 35 // MPANL at this frequency (defined on the protocol page)
result.mpanlsData[0].att = 30.6 // attenuation at this frequency (defined on the protocol page)
result.mpanlsData[0].levelUnderWahts = "11.3" // level under the ear cup (equal to level-att)
result.mpanlsData[0].noiseFloor = 41.5 // dosimeter noise floor at this frequency (dB SPL)
Schema
Modified Rhyme Test Response Area
A custom input area optimized for the Modified Rhyme test (MRT).
Protocol Example
{
"id": "MRT",
"title": "MRT Response Area Example",
"questionMainText": "Select the requested word",
"responseArea": {
"type": "mrtResponseArea",
"choices": [
{
"id": "Mark"
},
{
"id": "Bark"
},
{
"id": "Lark"
},
{
"id": "Dark"
},
{
"id": "Park"
},
{
"id": "Hark"
}
]
}
}
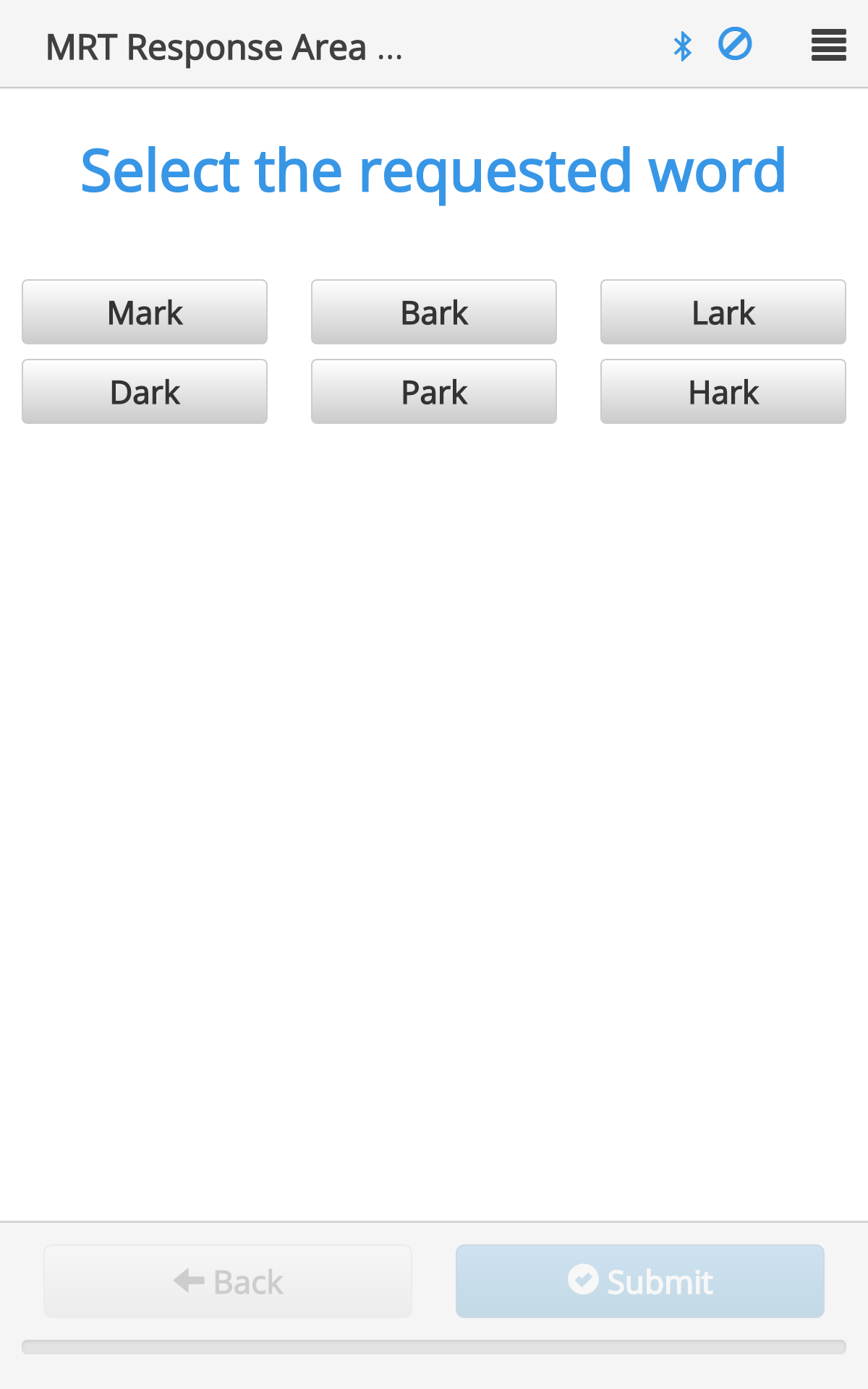
Options
choices
:- Type:
array
- Description: Array of objects containing the choice properties. The array must contain 6
choices
.
- Type:
verticalSpacing
:- Type:
integer
- Description: Vertical spacing between buttons, given in [px].
- Type:
horizontalSpacing
:- Type:
integer
- Description: Horizontal spacing between buttons, given in [px].
- Type:
feedback
:- Type:
string
- Description: Type of feedback to provide after submit. Can be
gradeResponse
(will mark correct/incorrect answers in green/red) orshowCorrect
(will grade AND show the correct choice).
- Type:
Response
The result.response
from an mrtResponseArea
is a string corresponding to the id
of the selected button. Note that the text
displayed on the button is not necessarily the same as the id
of the button (see Choice Properties). If the user selected the button choice with id
of Park
:
result.response = "Park"
Additionally, if one of the options is identified as correct
within the page definition, result.correct
will indicate if the user responded correctly.
Schema
Multiple Choice Response Area
Allows selection of a single item from an arbitrary number of radio button choices.
Protocol Example
{
"id": "Multiple Choice",
"title": "Multiple Choice Response Area Example",
"questionMainText": "Select Next Action",
"responseArea": {
"type": "multipleChoiceResponseArea",
"choices": [
{
"id": "Questionnaire"
},
{
"id": "Adaptive Questions"
},
{
"id": "Audio Questions"
},
{
"id": "Finish and Submit"
}
],
"other": "Something Else"
}
}
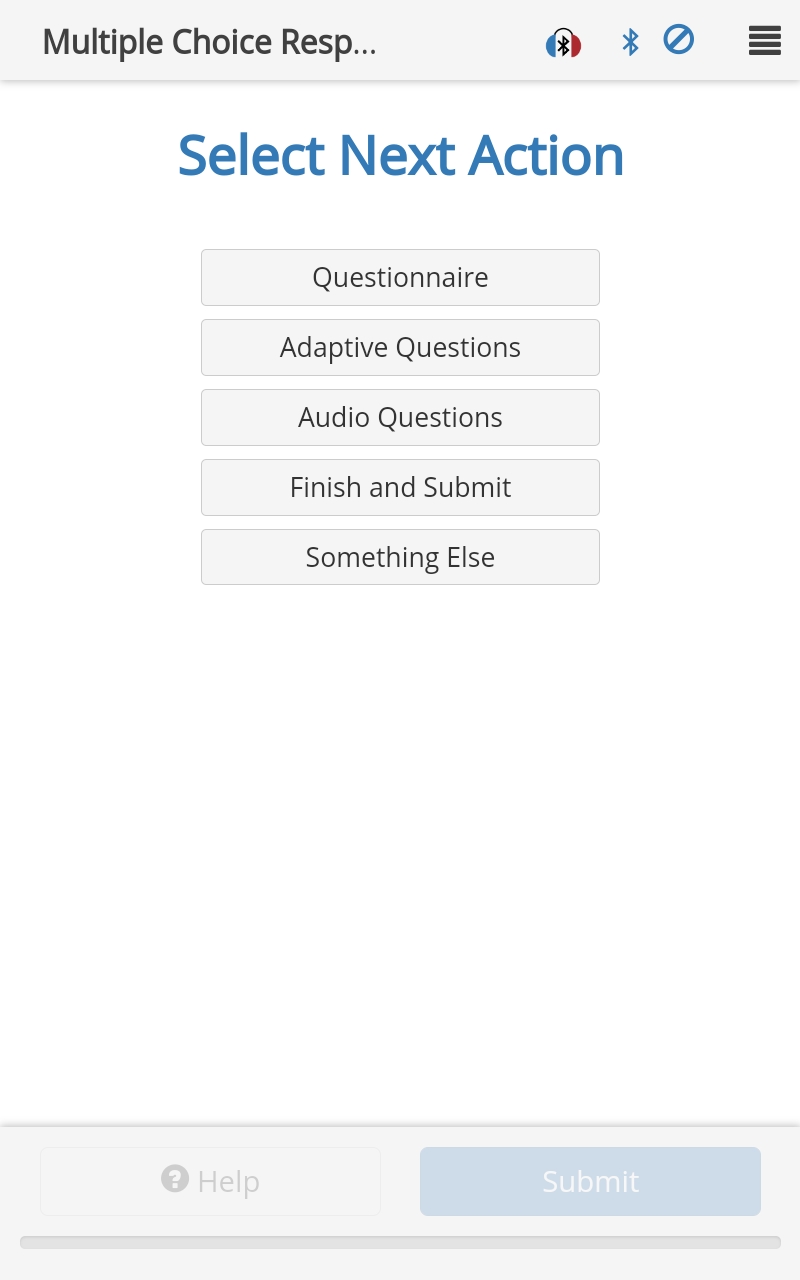
Options
choices
:- Type:
array
- Description: Array of objects containing the choice properties. The array must contain 6 objects.
- Type:
other
:- Type:
string
- Description: Text label for an 'other' choice. E.g.,
Other
, orSomewhere else
. If selected, the user will be prompted for an optional text value which will be stored in anotherResponse
field in the result object. If this field is not defined, theOther
choice is not presented.
- Type:
verticalSpacing
:- Type:
integer
- Description: Vertical spacing between buttons, given in [px].
- Type:
delayEnable
:- Type:
integer
- Description: Delay (ms) before the buttons are active to accept a response. (Default =
0
)
- Type:
exportToCSV
:- Type:
boolean
- Description: Whether results for this page should be exported to CSV upon submitting exam results
- Type:
Response
The result.response
from a multipleChoiceResponseArea
is either a string corresponding to the id
of the button selected or the string Other
(if the button defined as other
is chosen). In this protocol example, if the user were to select the button labeled Something Else
and then enter Test
in the text box when prompted:
result.response = "Other"
result.otherResponse = "Test"
Schema
Multiple Choice Selection Response Area
Presents a matrix of selections, where the user selects one item from each column.
Protocol Example
{
"id": "multipleChoiceSelection",
"title": "Multiple Choice Selection",
"questionMainText": "Multiple Choice Selection",
"questionSubText": "Page is submittable after each column has a response. You will see whether your responses are correct or incorrect.",
"responseArea": {
"type": "multipleChoiceSelectionResponseArea",
"rows": [["test", "test", "test"], ["three", "three", "three"], ["and", "four", "rows"], ["long", "for", "testing"]],
"columnLabels": ["My"," Column", "Labels"],
"correct": "test four testing",
"feedback": "showCorrect"
}
}
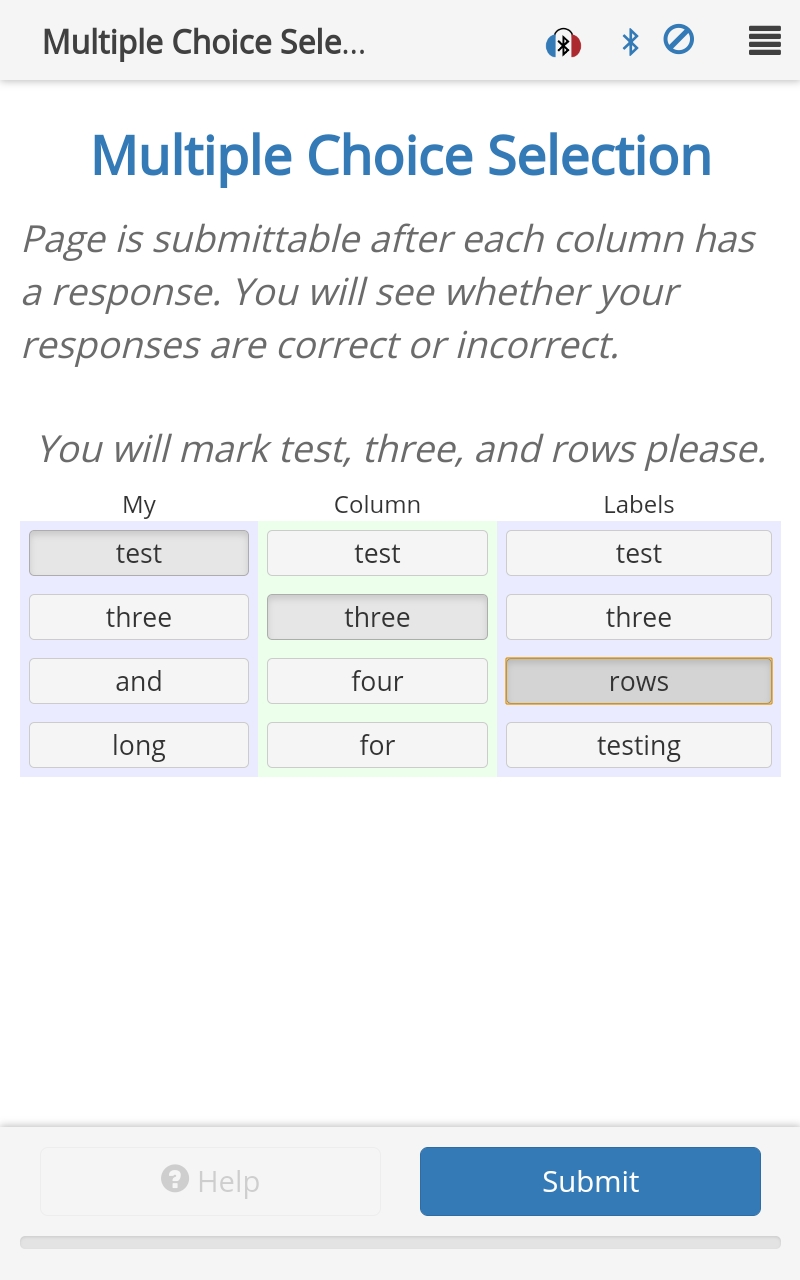
Options
columnLabels
:- Type:
string array
- Description: Labels for columns. The number of columns must match the number of columns in
rows
. The number of columns must be between 2 and 5.
- Type:
rows
:- Type:
2D string array
- Description: n x m array. The number of rows (n) must be between 1 and 10. The number of columns (m) must match the number of elements in the
columnLabels
array. If not specified, defaults to standard 10 x 5 OMT array.
- Type:
autoSubmit
:- Type:
boolean
- Description: If
true
, go straight to next page when a choice is selected. (Default =false
)
- Type:
correct
:- Type:
string
- Description: The correct answer (e.g.
Alan bought some big beds
). It must have the same number of words as there are columns in the word matrix.
- Type:
feedback
:- Type:
string
- Description: Provide feedback after submit. Can be
gradeResponse
(will mark answers correct/incorrect in green/red) orshowCorrect
(will grade AND show the correct choice).
- Type:
Response
The result.response
from a multipleChoiceSelectionResponseArea
is a string containing the responses selected from each column concatenated together with a space delimiter. Additional result fields indicate if the response(s) were correct. For example:
result.response = "test three rows"
result.eachCorrect = [true, false, false] // array of boolean values with one value given per column
result.correct = false // true if all column responses are correct, false otherwise
result.numberCorrect = 1 // number of correct column responses
result.numberIncorrect = 2 // number of incorrect column responses
Schema
Multiple Input Response Area
Allows multiple inputs of different types on the same page.
Protocol Example
{
"id": "multiline",
"title": "Multiple Input Response Area",
"questionMainText": "Enter the values associated with this test",
"questionSubText": "Confirm that the dropdown menus work and that some of the text boxes can be populated.",
"responseArea": {
"type": "multipleInputResponseArea",
"verticalSpacing": 15,
"textAlign": "left",
"inputList": [
{
"text": "Subject Age"
},
{
"text": "Subject Id"
},
{
"text": "Test Location"
},
{
"text": "Administrator Name"
},
{
"inputType": "number",
"text": "Numerical Input"
},
{
"inputType": "dropdown",
"options": [1,2,3,4,5,6,7,8,9],
"text": "Dropdown Input"
},
{
"text": "Other important values"
}
]
}
}
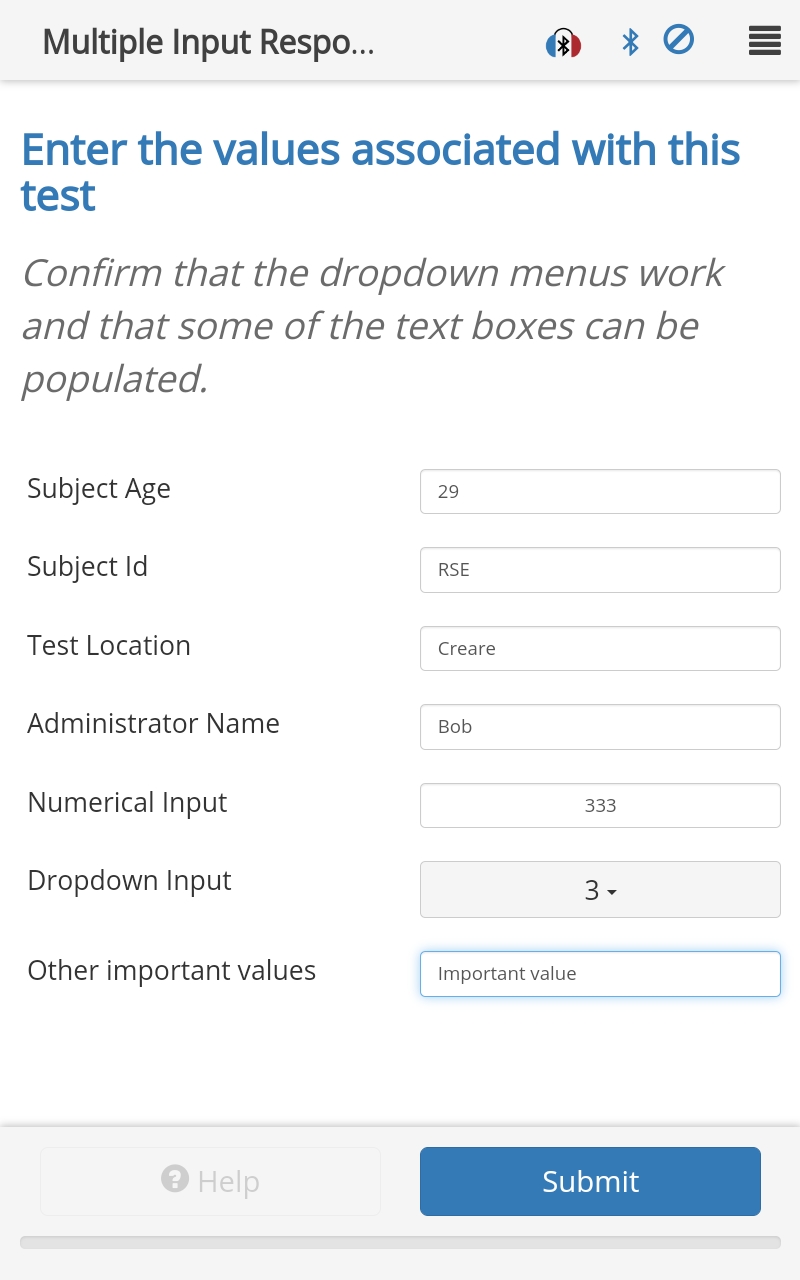
Options
choices
:- Type:
array
- Description: Array of objects containing the choice properties.
- Type:
inputTypeForAll
:- Type:
string
- Description: Set input type for the entire list. This value can be overridden by individual inputs. Can be
text
,number
,dropdown
, ormulti-dropdown
.
- Type:
verticalSpacing
:- Type:
integer
- Description: Vertical spacing between buttons, given in [px].
- Type:
textAlign
:- Type:
string
- Description: Horizontal alignment of text labels. Can be
left
,right
orcenter
. (Default =center
)
- Type:
review
:- Type:
boolean
- Description: If
true
, allows the inputs to be reviewed without the input boxes.(Default =false
)
- Type:
inputList
:Type:
array
Description: An array of objects containing the following:
inputType
:- Type:
string
- Description: Type of input, based on html input type. Can be
text
,number
,dropdown
,date
, ormulti-dropdown
. (Default =text
)
- Type:
dateProperties
:Type:
object
Description: Optional properties for when the
inputType
is adate
.maxDate
:- Type:
string
- Description: Maximum date allowed in ISO formatted string YYYY-MM-DD or
today
- Type:
minDate
:- Type:
string
- Description: Minimum date allowed in ISO formatted string YYYY-MM-DD or
today
- Type:
text
:- Type:
string
- Description: Label for this input object.
- Type:
options
:- Type:
array
- Description: Array of options used when the
inputType
isdropdown
ormulti-dropdown
.
- Type:
required
:Type:
boolean
Description: If
true
, the page is not submittable without a response for this input. (Default =false
)exportToCSV
:Type:
boolean
Description: If
true
, export the result to CSV upon submitting exam results. (Default =false
)
Response
The result.response
from a multipleInputResponseArea
is an array of length equal to the number of input selections presented. The type of each individual array element is governed by the inputType
defined. A text
or date
input results in a string response. A number
input provides a numeric response. Responses to a dropdown
type input are governed by the dropdown options presented (for the example given here the result would be numeric but if the dropdown options were strings then the result would be a string).
Schema
NATO Response Area
NATO input area.
Protocol Example
{
"id": "NATO",
"questionMainText": "Please read the following sentence",
"responseArea": {
"type": "natoResponseArea",
"sentence": "Kathy has four cheap rings",
"microphone": "internal"
}
}
Options
sentence
:- Type:
string
- Description: Sentence to be displayed to user. Required.
- Type:
microphone
:- Type:
string
- Description: Microphone to use for recording. Can be
internal
orexternal
. (Default =internal
)
- Type:
autoPlay
:- Type:
boolean
- Description: If
true
, automatically play the recorded audio once recording is stopped. (Default =true
)
- Type:
Schema
Oldenburg Matrix Test Response Area
Allows for the Oldenburg Matrix Test (OMT) to be administered.
Protocol Example
{
"id": "omt",
"title": "OMT Response Area",
"questionMainText": "OMT Response Area",
"responseArea": {
"type": "omtResponseArea",
"correct": "Alan bought some big beds."
}
}
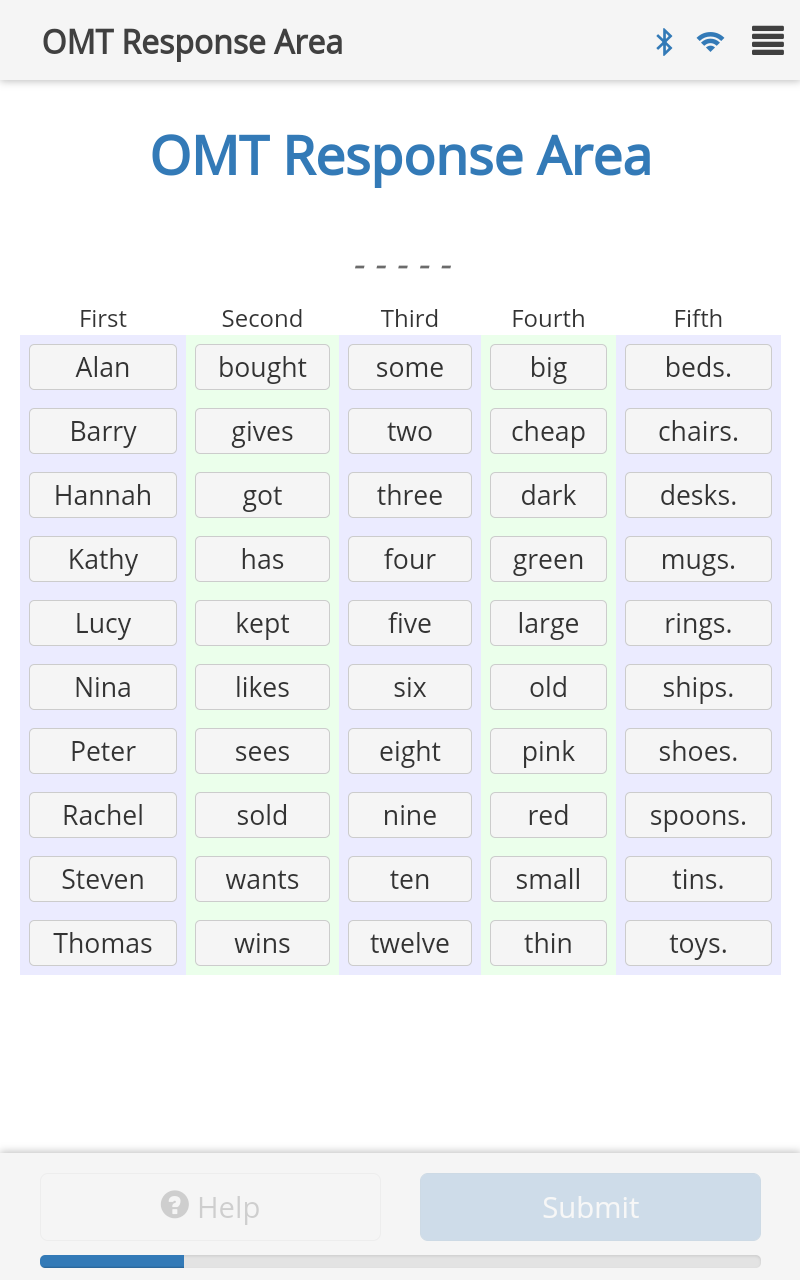
Options
language
:- Type:
string
- Description: Determines which set of words are used, from International Matrix Tests. Unused if 'rows' property is specified.",
- Type:
autoSubmit
:- Type:
boolean
- Description: If
true
, go straight to next page when a choice is selected. (Default =false
)
- Type:
correct
:- Type:
string
- Description: Correct answer, such as 'Alan bought some big beds'.
- Type:
feedback
:- Type:
string
- Description: Provide feedback after submit. Can be
gradeResponse
orshowCorrect
.
- Type:
Response
The result.response
from a omtResponseArea
is a string containing the responses selected from each column concatenated together with a space delimiter.
result.response = "Alan bought some big beds." // String of the selected words
Schema
QR Response Area
Use to import data by scanning a QR code.
Protocol Example
{
"id": "qr001",
"title": "QR Code Support",
"questionMainText": "Please scan in the appropriate QR code.",
"questionSubText": "QR input can be set to mandatory, or can be set to allow the user to continue without scanning a QR code.",
"responseArea": {
"type": "qrResponseArea",
"responseRequired": false
}
}
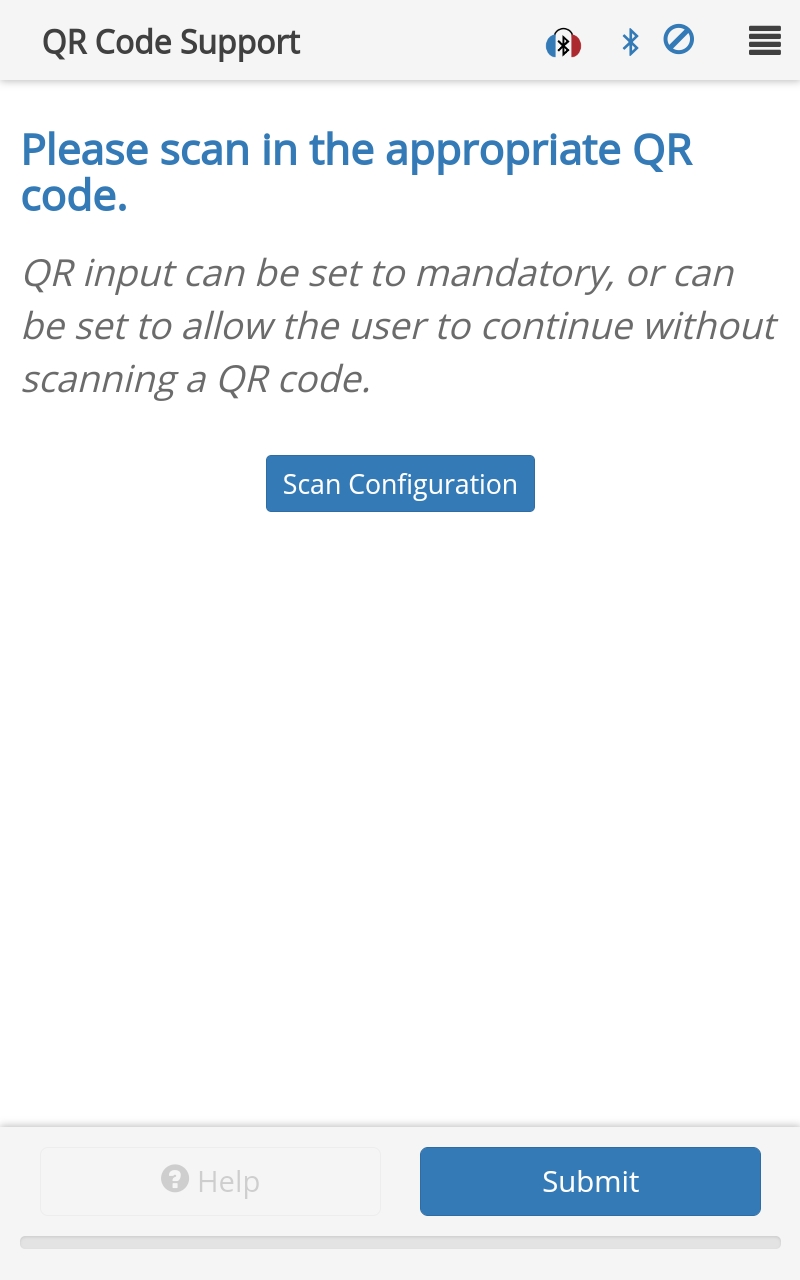
Options
scope
:- Type:
string
- Description: Can be
exam
orpage
. Ifexam
, the scanned QR code sets the single exam-wide QR code. Ifpage
, the scanned QR code is only recorded as an answer for this page.
- Type:
autoSubmit
:- Type:
boolean
- Description: If
true
, automatically submit the page when the QR code is scanned. (Default =true
)
- Type:
Response
The result.response
from a qrResponseArea
is a string. The value of the string is determined by the information contained within the QR code.
Schema
Results View Response Area
Allows the user to view and save certain results from specified exams in a PDF document. Only results from a Multiple Input Response Area, Text Box Response Area, MPANL Response Area, Likert Response Area, Three Digit Response Area, Audiometry Results Plot Response Area, and Audiometry Results Table Response Area can be displayed.
The results for each object within displayResults
will be collapsed under the title heading and can be expanded to view the results by clicking on the heading. After viewing the results, check the Save Copy as PDF
box before submitting the page in order to export the results view as a PDF. All sections will automatically be expanded to show all results in the exported file.
See the Results View Example Protocol for a full example of how to use it within a protocol.
Protocol Example
{
"id": "results_view",
"title": "Results View",
"questionMainText": "Basic Audiometry Results",
"instructionText": "Review and select Submit to Export as PDF",
"responseArea": {
"type": "resultsViewResponseArea",
"displayResults": [
{
"text": "Subject Info",
"displayIds": ["subject_info"]
},
{
"text": "Comments",
"displayIds": ["comments"]
},
{
"text": "Three Digit",
"displayIds": ["threeDigit_practice"]
},
{
"text": "Likert",
"displayIds": ["likert001"]
},
{
"text": "chaBekesyLike",
"displayIds": ["bekesy_like_table"]
},
{
"text": "chaHughsonWestlake",
"displayIds": ["bekesy_like_table"]
},
{
"text": "chaManualAudiometry",
"displayIds": ["ManualAudiometryTable", "ManualAudiometryPlot"]
},
{
"text": "chaBHAFT",
"displayIds": ["bhaft_table"]
},
{
"text": "chaMLD",
"displayIds": ["mld_table"]
},
{
"text": "MPANL",
"displayIds": ["MPANL"]
},
{
"text": "Automated Screener",
"displayIds": ["autoscreen_results"]
}
]
}
}
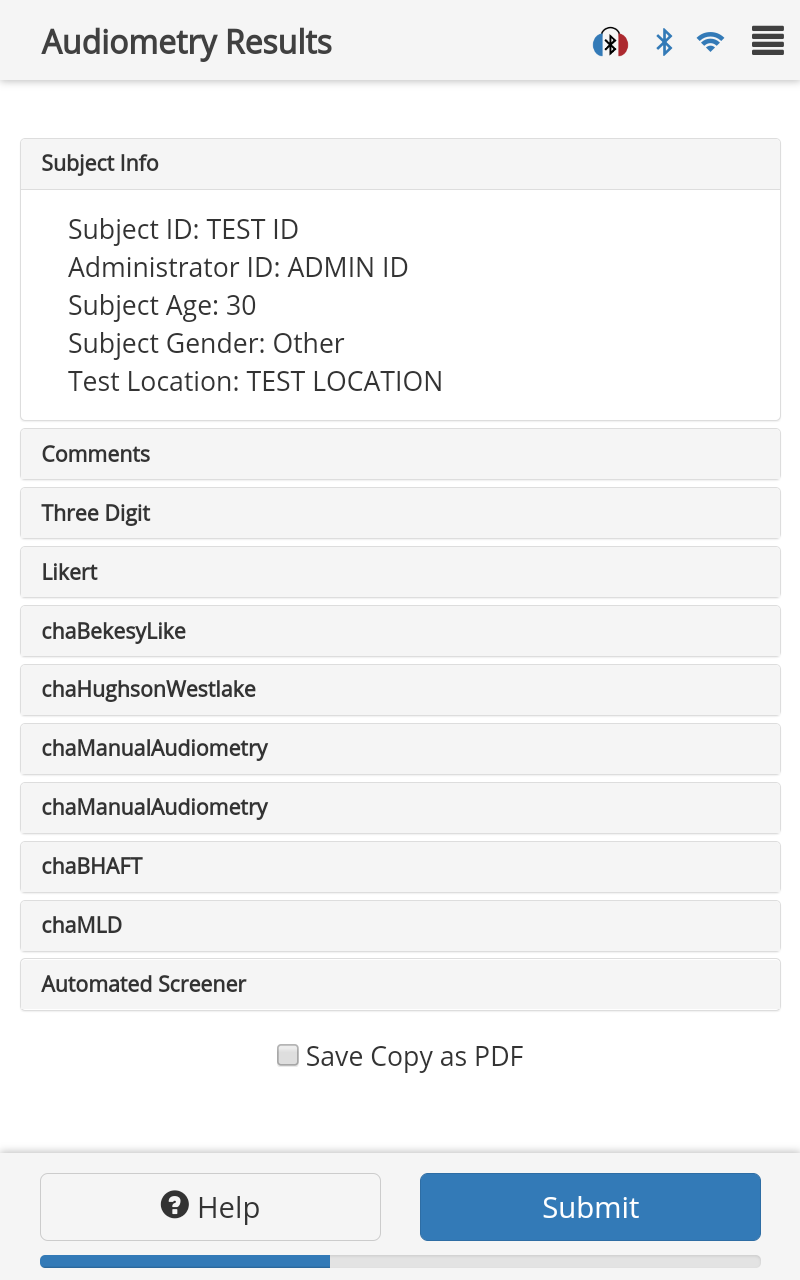
Options
displayResults
:Type:
array
Description: An array of objects with page
id
's and display names to include in the results view.text
:- Type:
string
- Description: Title of Results View section.
- Type:
displayIds
:- Type:
array
- Description: Array of page
id
's to include in this section.
- Type:
Response
The resultsViewResponseArea
saves a PDF document in the Documents/tabsint-pdfs
folder of the internal storage of the tablet. The Admin PIN must be entered to open the file.
Schema
SeeSaw Response Area
Allows user to indicate a desired trade-off between two sets of criteria.
Protocol Example
{
"id": "SeeSaw",
"title": "Weighing Options",
"questionMainText": "Protection vs. Distortion",
"questionSubText": "Response required to submit page.",
"responseArea": {
"type": "seeSawResponseArea",
"leftHeader": "How well my HPD protects me from loud impulse sounds like gunfire or explosions",
"rightHeader": "How natural my own voice sounds while talking when wearing my HPD",
"leftLabels": [
"Much less protection",
"Somewhat less protection",
"No change",
"Somewhat more protection",
"Much more protection"
],
"rightLabels": [
"Much more distortion",
"Somewhat more distortion",
"No change",
"Somewhat more natural sound",
"Much more natural sound"
]
}
}
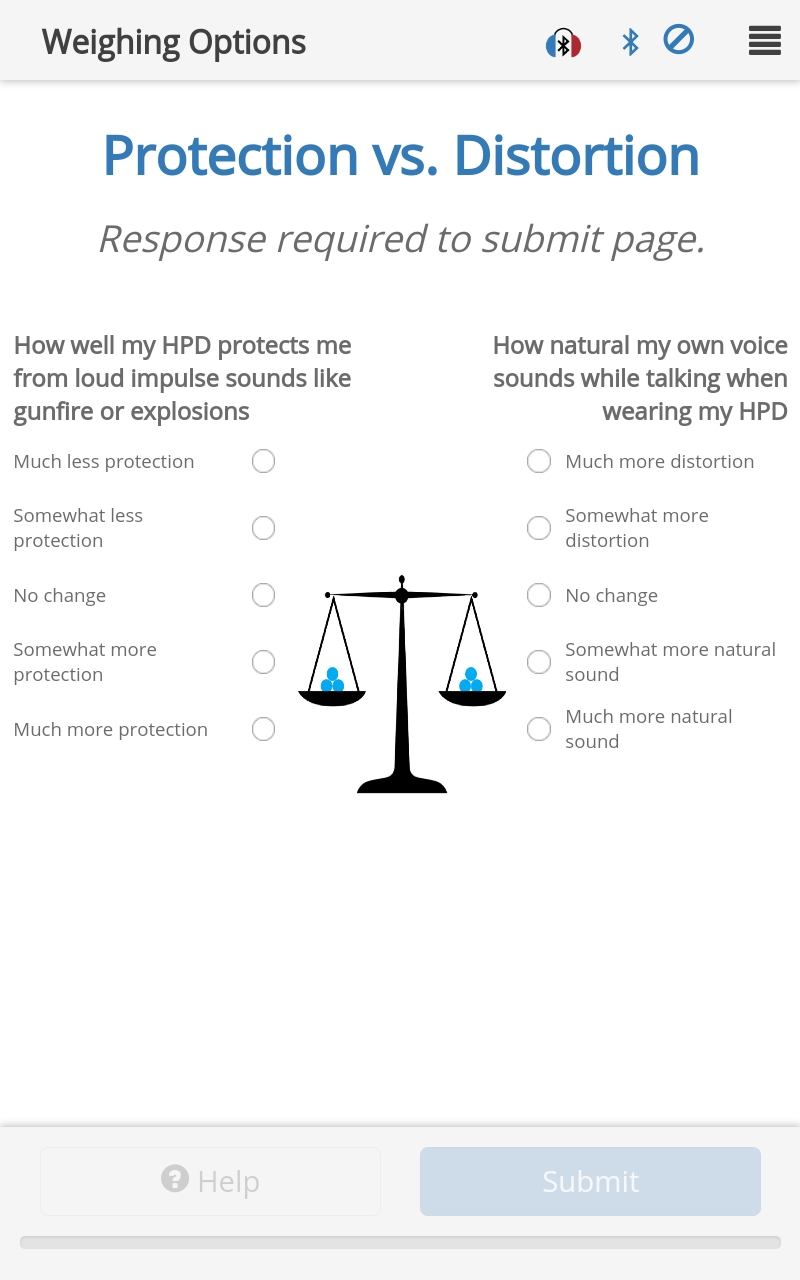
Options
leftHeader
:- Type:
string
- Description: Header above choices on the left side of the response area.
- Type:
leftLabels
:- Type:
string array
- Description: Five labels for the left side of the response area.
- Type:
rightHeader
:- Type:
string
- Description: Header above choices on the right side of the response area.
- Type:
rightLabels
:- Type:
string array
- Description: Five labels for the right side of the response area.
- Type:
Response
The result.response
from a seeSawResponseArea
is a number corresponding to the 0-based level selected on the left side. In this example, if the user selected "Somewhat more protection" on the left side then the result would be:
result.response = 3 // Note this is a number.
Schema
Subject ID Response Area
A response area to record a subject identification code in the exam results.
Protocol Example
{
"id": "Subject ID",
"title": "Subject Id Response Area",
"questionMainText": "Enter the Subject ID",
"questionSubText": "Enter the Subject ID manually. Require response to be defined before activating submit button on a page.",
"responseArea": {
"type": "subjectIdResponseArea"
}
}
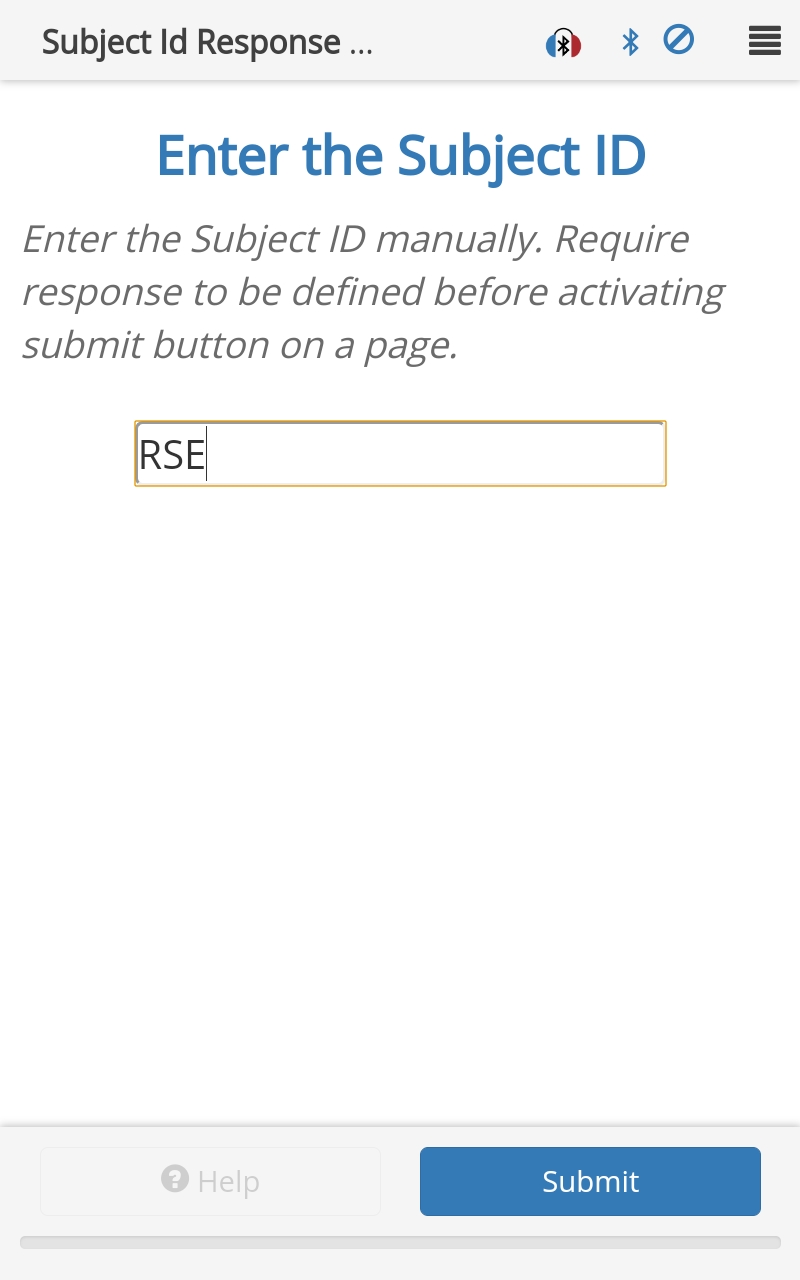
Options
generate
:- Type:
boolean
- Description: If
true
, the subject ID will be automatically generated. (Default =false
)
- Type:
Response
The result.response
from a subjectIdResponseArea
is a string corresponding to the text entered by the user. In this example, the result would be:
result.response = "RSE"
Schema
Text Box Response Area
Presents the user with text box for data entry.
Protocol Example
{
"id": "Text Box",
"title": "Text Box Response Area Example",
"questionMainText": "Enter your name",
"responseArea": {
"type": "textboxResponseArea",
"rows": 1
}
}
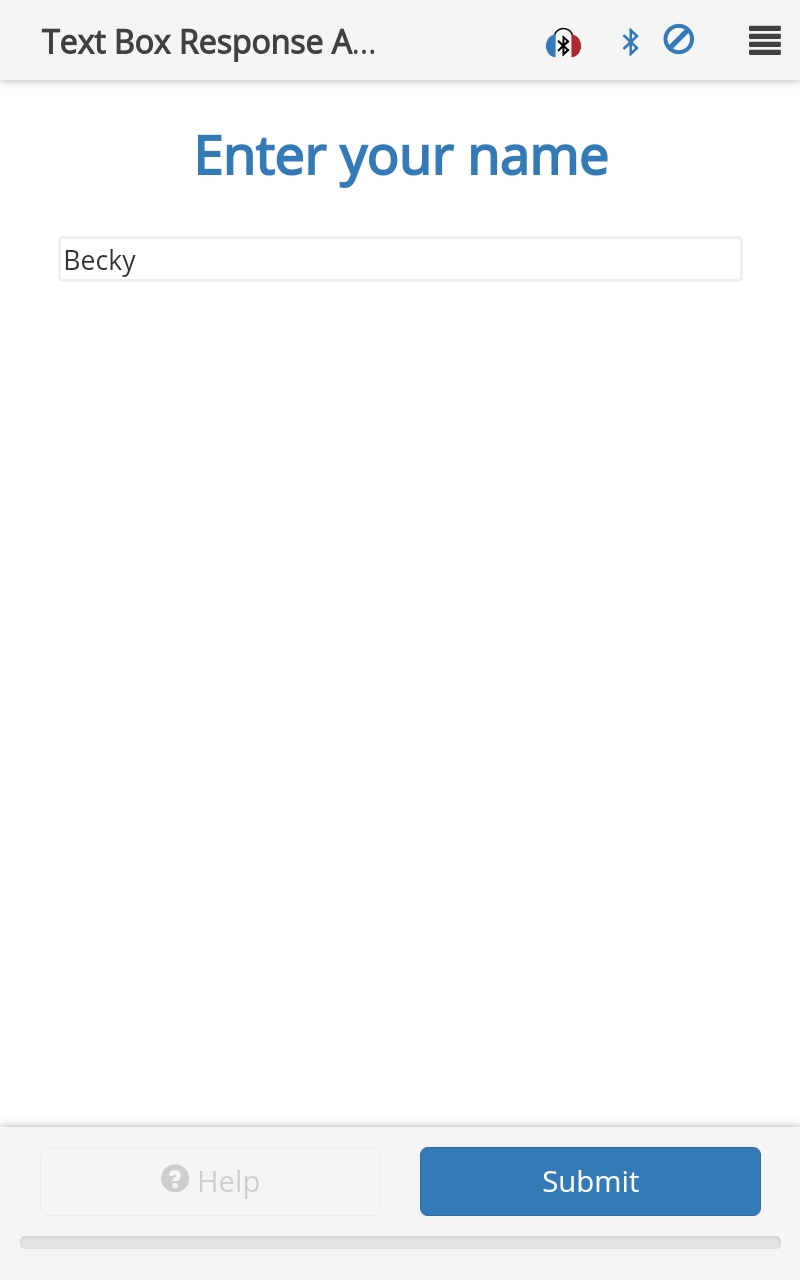
Options
rows
:- Type:
integer
- Description: Number of rows to display in the input box. (Default =
1
)
- Type:
exportToCSV
:- Type:
boolean
- Description: If
true
, export the result to CSV upon submitting exam results. (Default =false
)
- Type:
Response
The result.response
from a textboxResponseArea
is the string entered by the user in the text box.
Schema
Three Digit Test Response Area
This integer response area allows the input of a three digit number.
Protocol Example
{
"id": "Three Digit Answers",
"title": "Three Digit Test Response Area Example",
"questionMainText": "Enter the three digits heard",
"responseArea": {
"type": "threeDigitTestResponseArea"
}
}
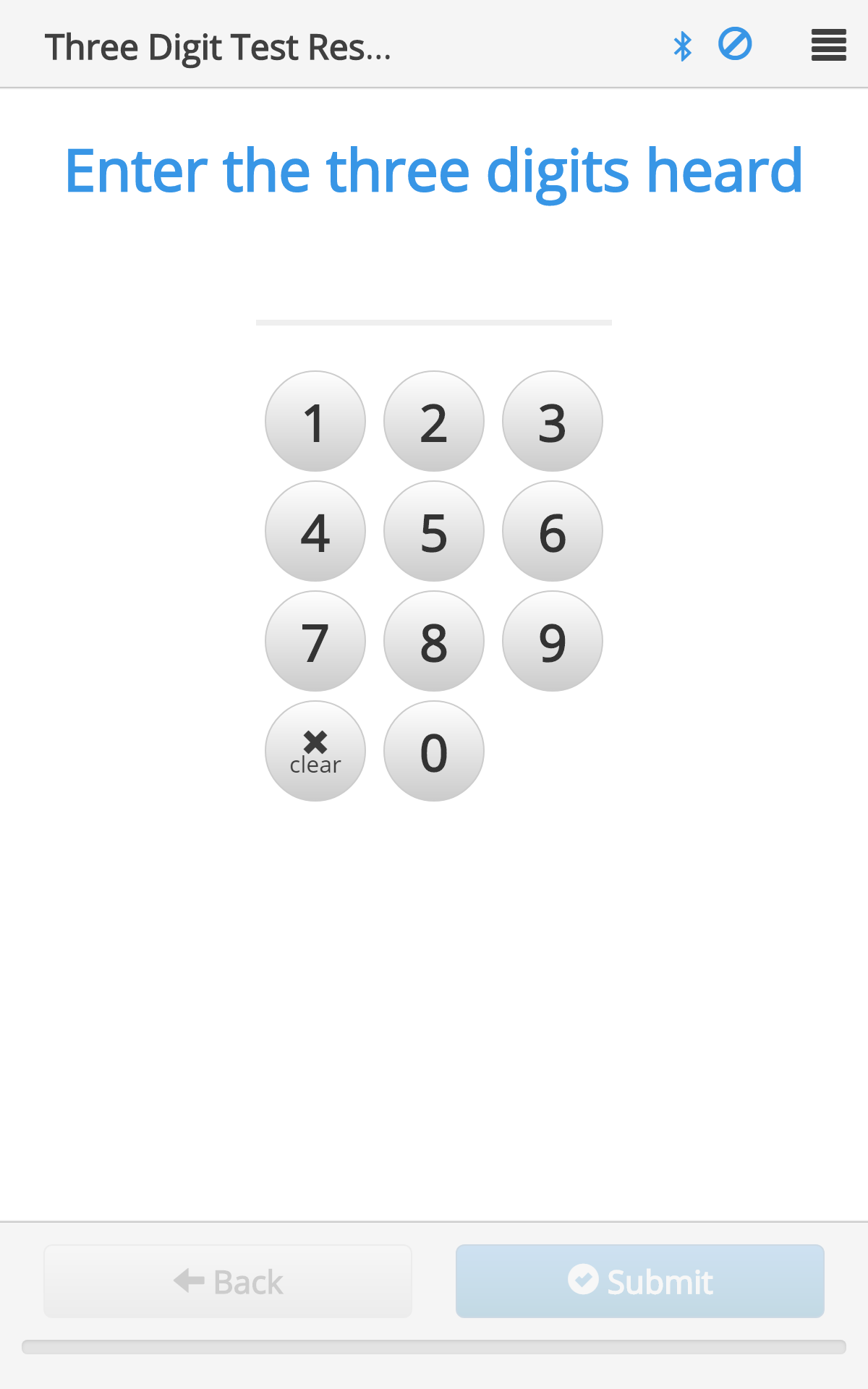
Options
correct
:- Type:
array of strings
- Description: The three correct answers in an array of strings.
- Type:
delayEnable
:- Type:
integer
- Description: Delay (ms) before the buttons are active to accept a response. (Default =
0
)
- Type:
Response
The result.response
from a threeDigitTestResponseArea
is a 3-element string array. The string values correspond to the numbers entered by the user in the order they were entered. For example:
result.response = ["1","5","9"] // Note this is a string array.
Schema
Yes-No Response Area
The Yes-No response area type is used to present the user with a simple yes or no question.
Protocol Example
{
"id": "yes-no-id",
"title": "Yes-No Response Area Example",
"questionMainText": "Is this a yes-no response area example?",
"questionSubText": "Select yes",
"responseArea": {
"type": "yesNoResponseArea"
}
}
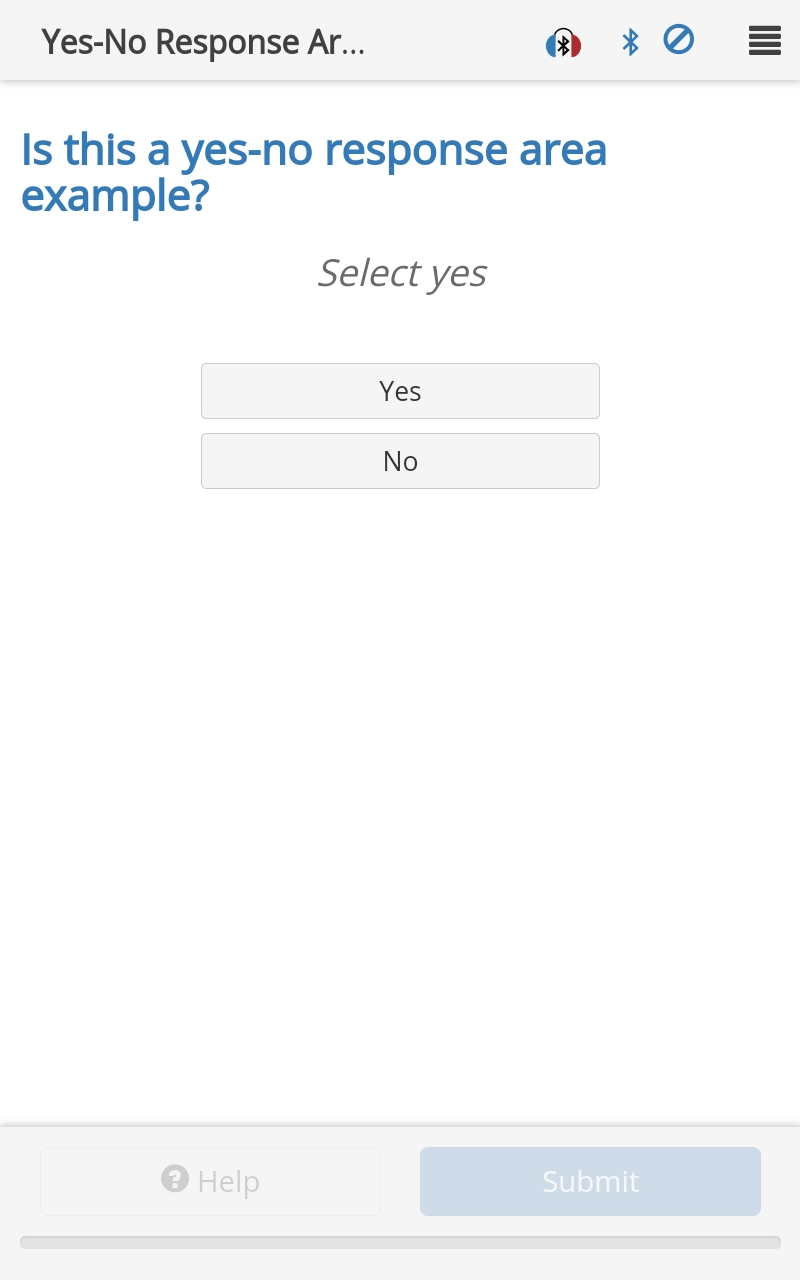
Options
verticalSpacing
:- Type:
integer
- Description: Vertical spacing between buttons, given in [px]
- Type:
Response
The result.response
from an yesNoResponseArea
is a string. If the user selected the button labeled Yes
the result would be:
result.response = "yes"
Schema
Common Response Area Properties
The following options can be used with any response area.
Protocol Example
{
"responseArea": {
"type": "any-response-areas",
"responseRequired": true,
"enableSkip": false
}
}
Options
responseRequired
:- Type:
boolean
- Description: Require response to be defined. Setting to
false
allows empty submission. (Default =true
)
- Type:
enableSkip
:- Type:
boolean
- Description: Show a
Skip
button that allows user to skip this question. (Default =false
)
- Type:
Schema
Choice Properties
The following options can be used with any response area involving multiple choices (button grid response area, checkbox response area, MRT response area, multiple choice response area).
Options
id
:- Type:
string
- Description: A string representing this choice, as it will be recorded in the database. Required.
- Type:
text
:- Type:
string
- Description: User-facing text; can be more verbose than
id
. Defaults to contents ofid
.
- Type:
correct
:- Type:
boolean
- Description:
true
if this is the correct response. If no choices are correct, then no scoring is performed.
- Type:
disable
:- Type:
boolean
- Description: If
true
, the button is disabled. (Default =false
)
- Type:
textColor
:- Type:
string
- Description: Specify button text color e.g.
#FF0000
for red orgreen
for color green. (Default =tabsint button text color
)
- Type:
backgroundColor
:- Type:
string
- Description: Specify button background color e.g.
#FF0000
for red orgreen
for color green. (Default =tabsint button background color
)
- Type:
fontSize
:- Type:
string
- Description: Specify button text size in pixels e.g.
20px
. (Default =tabsint button button text size
)
- Type:
Schema